Simple and Efficient Database Management with MongoDB

- Published on
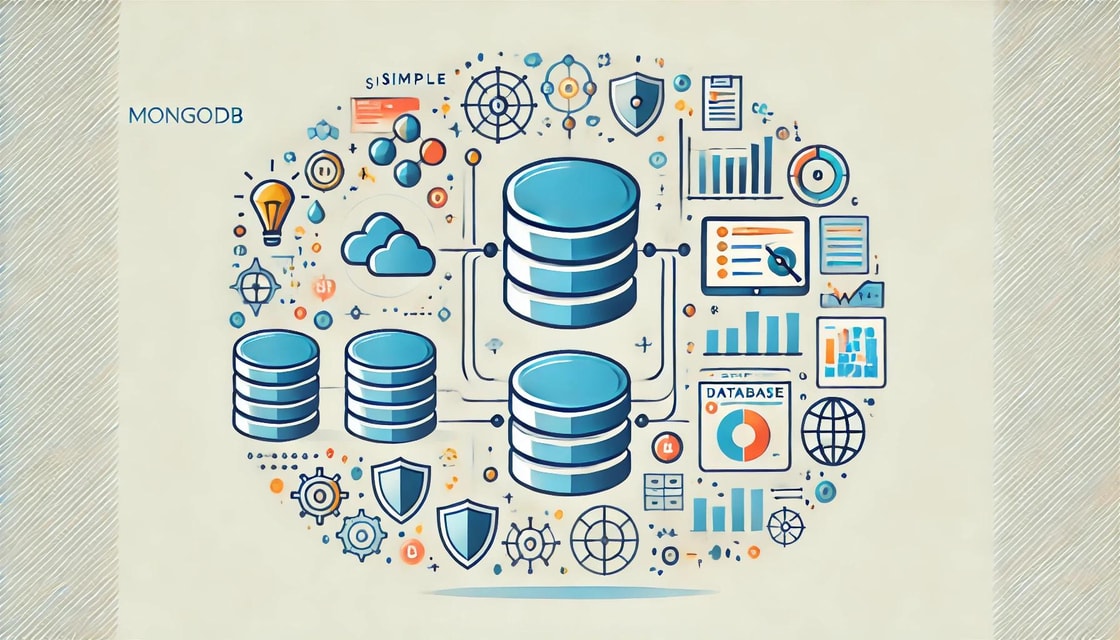
MongoDB is a powerful NoSQL database that provides flexibility and scalability for managing data. It is widely used in modern web applications due to its ability to handle large volumes of unstructured data and its ease of use.
Why MongoDB Matters
MongoDB is designed to handle large datasets and offers a flexible schema model that allows for rapid development and iteration. Its scalability and performance make it an ideal choice for modern applications that require efficient data management and real-time analytics.
Getting Started with MongoDB
To start using MongoDB, you need to set up a MongoDB server and connect to it from your application. MongoDB provides various tools and libraries to make this process straightforward.
Installing MongoDB
You can install MongoDB on your local machine or use a cloud service like MongoDB Atlas. Here are the steps to install MongoDB locally:
- Download MongoDB from the official website.
- Install MongoDB by following the instructions for your operating system.
- Start the MongoDB server by running
mongod
.
Connecting to MongoDB
Use a MongoDB client or library to connect to your MongoDB server. For example, using the MongoDB Node.js driver:
const { MongoClient } = require('mongodb');
const uri = "mongodb://localhost:27017";
const client = new MongoClient(uri, { useNewUrlParser: true, useUnifiedTopology: true });
async function run() {
try {
await client.connect();
console.log("Connected to MongoDB");
} finally {
await client.close();
}
}
run().catch(console.dir);
Managing Data with MongoDB
MongoDB allows you to perform various operations to manage your data efficiently, including CRUD (Create, Read, Update, Delete) operations.
Creating Documents
Documents in MongoDB are JSON-like objects stored in collections. You can create a new document using the insertOne
method:
async function createDocument(db) {
const collection = db.collection('users');
const result = await collection.insertOne({ name: "Alice", age: 25, city: "New York" });
console.log(`New document created with _id: ${result.insertedId}`);
}
Reading Documents
To read documents, use the find
method:
async function readDocuments(db) {
const collection = db.collection('users');
const cursor = collection.find({ city: "New York" });
await cursor.forEach(console.log);
}